1. Message Oriented Communication and Resource Oriented Communication
➤ Message Oriented Communication
- Message-oriented communication is a way of communicating between processes.
- Messages, which correspond to events, are the basic units of data delivered.
- Tanenbaum and Steen classified message-oriented communication according to two factors synchronous or asynchronous communication, and transient or persistent communication.
- In synchronous communication, the sender blocks waiting for the receiver to engage in the exchange.
- Asynchronous communication does not require both the sender and the receiver to execute simultaneously.
- So, the sender and recipient are loosely-coupled.
- The amount of time messages are stored determines whether the communication is transient or persistent.
- Transient communication stores the message only while both partners in the communication are executing.
- If the next router or receiver is not available, then the message is discarded.
- Persistent communication, on the other hand, stores the message until the recipient receives it.
➤ Resource Oriented Communication
- Resource oriented communication is a communication that aims to exploit the low-level communication facilities of today’s cluster networking hardware and to merge, via the resource oriented paradigm, those facilities and the high-level degree of parallelism achieved on SMP systems through multi-threading.
- The communication model defines three major entities – contexts, resources and buffers – which permit the design of high-level solutions.
- A low-level distributed directory is used to support resource registering and discovering.
- The usefulness and applicability of RoCL is briefly addressed through a basic modelling example – the implementation of TPVM over RoCL.
- Performance results for Myrinet and Gigabit Ethernet, currently supported in RoCL through GM and MVIA, respectively, are also presented.
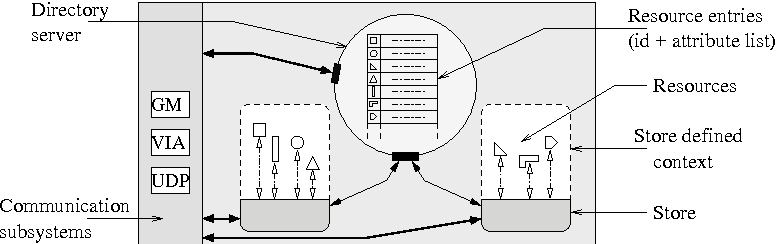
2. The Resource Based Nature of The REST Style

- The fundamental concept in any RESTful API is the resource. A resource is an object with a type, associated data, relationships to other resources, and a set of methods that operate on it. It is similar to an object instance in an object-oriented programming language, with the important difference that only a few standard methods are defined for the resource (corresponding to the standard HTTP GET, POST, PUT and DELETE methods), while an object instance typically has many methods.
- Resources can be grouped into collections. Each collection is homogeneous so that it contains only one type of resource, and unordered. Resources can also exist outside any collection. In this case, we refer to these resources as singleton resources. Collections are themselves resources as well.
- Collections can exist globally, at the top level of an API, but can also be contained inside a single resource. In the latter case, we refer to these collections as sub-collections. Sub-collections are usually used to express some kind of “contained in” relationship. We go into more detail on this in relationships.
- Resources have data associated with them. The richness of data that can be associated with a resource is part of the resource model for an API. It defines for example the available data types and their behavior.
- A RESTful approach to software development can greatly simplify the task of application integration, but this new approach can often cause troubles for those with more of a legacy background in distributed computing. That’s when developers finds themselves creating more problems than they fix. Louvel’s advice is not to take the usual, traditional approach when developing RESTful APIs. Forget what you learned from the service-oriented, RPC based world. Then, you’ll discover that you are working without limitations and you can really start to solve your business problems. Furthermore, the modularity involved in developing a RESTful architecture means developers have smaller, more agile parts that provide greater flexibility when designing solutions that really work.
3. Representations in REST Style
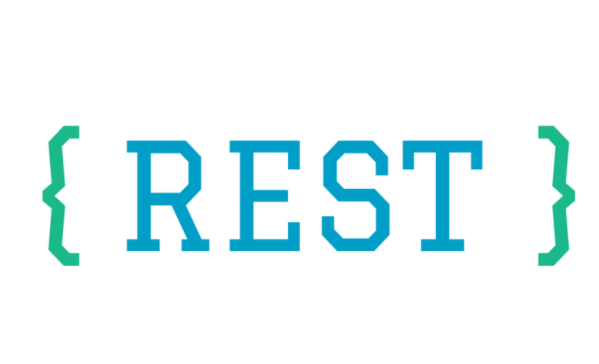
- Representation is the value/response of the Resource at a point in time based on the media-type.
- Resources can be manipulated only through their representations.
- This is exactly how browsing the web works. An HTML page is not a resource, it’s just one representation.
- And when we submit a form, we’re just sending a different representation back to the server.
- One resource could have many representations. Heck, you could get crazy and have an API where you’re able to request the XML, JSON or HTML representations of any resource.
- We’re just crazy enough that we’ll do some of that.
- A representation is a machine readable explanation of the current state of a resource.
- In REST-speak, a client and server exchange representations of a resource, which reflect its current state or its desired state.
- REST, or Representational state transfer, is a way for two machines to transfer the state of a resource via representations.
4. Constraints of REST
- REST stands for Representational State Transfer, a term coined by Roy Fielding in 2000. It is an architecture style for designing loosely coupled applications over HTTP, that is often used in the development of web services. REST does not enforce any rule regarding how it should be implemented at lower level, it just put high level design guidelines and leave you to think of your own implementation.
- REST defines 6 architectural constraints which make any web service – a true RESTful API.
➤ Uniform Interface
- As the constraint name itself applies, you MUST decide APIs interface for resources inside the system which are exposed to API consumers and follow religiously. A resource in the system should have only one logical URI and that should provide a way to fetch related or additional data. It’s always better to synonymise a resource with a web page.
- Any single resource should not be too large and contain each and everything in its representation. Whenever relevant, a resource should contain links (HATEOAS) pointing to relative URIs to fetch related information.
- Also, the resource representations across system should follow certain guidelines such as naming conventions, link formats or data format (xml or/and json).
- All resources should be accessible through a common approach such as HTTP GET and similarly modified using a consistent approach.
➤ Client-Server
- This essentially means that client application and server application MUST be able to evolve separately without any dependency on each other. A client should know only resource URIs and that’s all. Today, this is normal practice in web development so nothing fancy is required from your side. Keep it simple.
➤ Stateless
- Roy fielding got inspiration from HTTP, so it reflects in this constraint. Make all client-server interaction stateless. Server will not store anything about latest HTTP request client made. It will treat each and every request as new. No session, no history.
- If client application needs to be a stateful application for the end user, where user logs in once and do other authorized operations thereafter, then each request from the client should contain all the information necessary to service the request – including authentication and authorization details.
➤ Cacheable
- In today’s world, caching of data and responses is of utmost important wherever they are applicable/possible. The webpage you are reading here is also a cached version of the HTML page. Caching brings performance improvement for client side, and better scope for scalability for a server because the load has reduced.
- In REST, caching shall be applied to resources when applicable and then these resources MUST declare themselves cacheable. Caching can be implemented on the server or client side.
➤ Layered System
- REST allows you to use a layered system architecture where you deploy the APIs on server A, and store data on server B and authenticate requests in Server C, for example. A client cannot ordinarily tell whether it is connected directly to the end server, or to an intermediary along the way.
➤ Code On Demand
- Well, this constraint is optional. Most of the time you will be sending the static representations of resources in form of XML or JSON. But when you need to, you are free to return executable code to support a part of your application e.g. clients may call your API to get a UI widget rendering code. It is permitted.
5. Different Types of Implementations For The Elements of REST Style
- The key aspect of REST is the state of the data elements, its components communicate by transferring representations of the current or desired state of data elements. REST identifies six data elements: a resource, resource identifier, resource metadata, representation, representation metadata, and control data, shown in the table below
➤ Resource Element
- Any information that can be named is a resource. A resource is a conceptual mapping to a set of entities not the entity itself. Such a mapping can change over time. In general, a RESTful resource is anything that is addressable over the Web.
- Example -- Title of a movie from IMDb, A Flash movie from YouTube, Images from Flickr etc
➤ Resource Identifier Element
- Every resource must have a name that uniquely identifies it. Under HTTP these are called URIs. Uniform Resource Identifier (URI) in a RESTful system is a hyperlink to a resource. It is the only means for clients and servers to exchange representations of resources.The relationship between URIs and resources is many to one. A resource can have multiple URIs which provide different information about the location of a resource.
- Example -- Standardized format of URI:scheme://host:port/path?queryString#fragmente.g:http://some.domain.com/orderinfo?id=123
➤ Resource Metadata
- This describes the resource. A metadata provides additional information such as location information, alternate resource identifiers for different formats or entity tag information about the resource itself.
- Example --Source link, vary
➤ Representation
- It is something that is sent back and forth between clients and servers. So, we never send or receive resources, only their representations. A representation captures the current or intended state of a resource. A particular resource may have multiple representations.
- Example -- Sequence of bytes, HTML document, archive document, image document.
➤ Control Data
- This defines the purpose of a message between components, such as the action being requested.
- Example -- If-Modified-Since, If-Match
6. How to Define the API of RESTful Web Services Using RESTful URLs
- REST is used to build Web services that are lightweight, maintainable, and scalable in nature. A service which is built on the REST architecture is called a RESTful service. The underlying protocol for REST is HTTP, which is the basic web protocol.
- A RESTful API breaks down a transaction to create a series of small modules. Each module addresses a particular underlying part of the transaction. This modularity provides developers with a lot of flexibility, but it can be challenging for developers to design from scratch. Currently, the models provided by Amazon Simple Storage Service, Cloud Data Management Interface and OpenStack Swift are the most popular.
- A RESTful API explicitly takes advantage of HTTP methodologies defined by the RFC 2616 protocol. They use GET to retrieve a resource; PUT to change the state of or update a resource, which can be an object, file or block; POST to create that resource ; and DELETE to remove it.
- Under REST principles, a URL identifies a resource. The following URL design patterns are considered REST best practices:
- URLs should include nouns, not verbs.
- Use plural nouns only for consistency (no singular nouns).
- Use HTTP methods (HTTP/1.1) to operate on these resources:
- Use HTTP response status codes to represent the outcome of operations on resources.
➤ URL Depth
- The resource/identifier/resource URL pattern is sufficient for full attribute visibility between any resources. Therefor, this URL depth is usually sufficient to support any arbitrary resource graph. If your URL design goes deeper than resource/identifier/resource, it may be evidence that the granularity of your API is too coarse.
➤ API Payload Format Encoding
- To interact with an API, the consumer needs to know how the payload is encoded. This is true regardless of how many encoding formats the endpoint supports.
- The three patterns of payload format encoding most frequently found in the wild are:
- HTTP headers (e.g. Content-Type: and Accept:)
- GET parameters (e.g. &format=json)
- resource label (e.g. /foo.json)
- Using HTTP headers to specifying payload format can be convenient, however unfortunately not all clients handle headers consistently. Using HTTP headers alone will create issues for buggy clients.
- Using GET parameters to specify format is another common pattern for specifying the encoding of API payloads. This results in slightly longer URLs than resource label technique, and can occasionally create problems with caching behavior of some proxy servers.
- Resource label specification of API payload format, such as /foo/{id}.json, are functionally equivalent to GET parameter encoding but without the (admittedly rare) proxy caching issues.
8. JAX-RS API

- JAX-RS is a Java API for RESTful Web Services (JAX-RS) is a Java programming language API spec that provides support in creating web services according to the Representational State Transfer (REST) architectural pattern. JAX-RS uses annotations, introduced in Java SE 5, to simplify the development and deployment of web service clients and endpoints.
- From version 1.1 on, JAX-RS is an official part of Java EE 6. A notable feature of being an official part of Java EE is that no configurations is necessary to start using JAX-RS. For non Java EE 6 environments a small entry in the web . xml deployment descriptoris required.
➤ Implementations
- Apache CXF, an open source Web service framework
- Jersey, the reference implementation from Sun (now Oracle)
- WebSphere Application Server from IBM
- WebLogic Application Server from Oracle
- Cuubez framework
- Codenvy's Implementation
- Java Application Framework optimized for Google App Engine, including a powerful RESTful engine and comprehensive Data Authorization model
9. Annotations in JAX-RS
➤ Path
- It identifies the URI path. It can be specified on class or method.
➤ PathParam
- Represents the parameter of the URI path.
➤ GET
- specifies method responds to GET request.
➤ POST
- specifies method responds to POST request.
➤ PUT
- specifies method responds to PUT request.
➤ HEAD
- specifies method responds to HEAD request.
➤ QueryParam
- represents the parameter of the query string of an URL.
➤ Produces
- defines media type for the response such as XML, PLAIN, JSON etc. It defines the media type that the methods of a resource class or MessageBodyWriter can produce.
➤ Consumes
- It defines the media type that the methods of a resource class or MessageBodyReader can produce.
➤ CookieParam
- represents the parameter of the cookie.
➤ FormParam
- represents the parameter of the form.
➤ OPTIONS
- specifies method responds to OPTIONS request.
10. Media Type in JAX-RS

- All resource methods can consume and produce content of almost any type. If you make a POST request to a URI, such as api/books , the REST API expects the HTTP body to contain a payload that represents the resource it should create.
- This resource can be represented using any media type. Although typically, it will be represented in either, JSON or XML , but ut could be plain text , binary or a custom format. It doesn't matter , as long as there is a method in the resource class that can consume that media type.
➤ javax.ws.rs.core
- Low-level interfaces and annotations used to create RESTful service resources.
➤ javax.ws.rs.ext
- APIs that provide extensions to the types supported by the JAX-RS API
No comments:
Post a Comment